How Laravel Has-Through Relationship Works
- Published on
- • 3 mins read•––– views
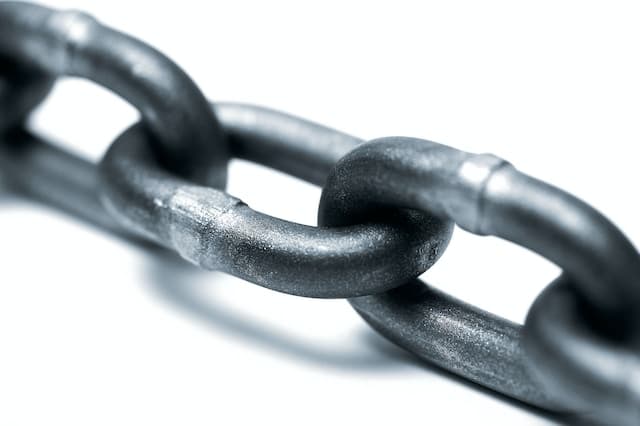
Introduction
Laravel Eloquent provides a powerful feature called the "has-through" relationship, which allows you to establish a direct relationship between two models through an intermediate model. This intermediate model serves as a bridge to connect the primary models without requiring a direct relationship between them.
In this article, we will explore how the "has-through" relationship works in Laravel and how it simplifies complex data retrieval scenarios.
Understanding the Relationship
Suppose we have three models: User
, Article
, and Category
.
- Each
User
can write multipleArticle's
. - Each
Article
can belong to multipleCategory's
.
The traditional approach would be to create direct relationships between User
and Article
, as well as between Article
and Category
. However, with the "has-through" relationship, we can avoid the need for a direct relationship between User
and Category
.
Defining the Relationships
Let's define the relationships in the model files:
User Model
class User extends Model
{
public function articles()
{
return $this->hasMany(Article::class);
}
}
Article Model
class Article extends Model
{
public function categories()
{
return $this->belongsToMany(Category::class);
}
public function user()
{
return $this->belongsTo(User::class);
}
}
Category Model
class Category extends Model
{
// Define other relationships or attributes for Category if needed.
}
The Has-Through Magic
Now, let's use the "has-through" relationship to retrieve the categories associated with articles written by a specific user without explicitly defining a direct relationship between User
and Category
.
use App\Models\User;
$user = User::find(1); // Replace 1 with the user's ID you want to retrieve articles for.
$categories = $user->articles->flatMap(function ($article) {
return $article->categories;
});
With the above code, we can access the categories related to articles written by the specified user. Laravel will automatically handle the intermediate steps to retrieve the data, making the process seamless and efficient.
Conclusion
The "has-through" relationship in Laravel Eloquent allows you to access related data through multiple layers of relationships without explicitly defining direct relationships between primary models. This powerful feature simplifies complex data retrieval scenarios and enhances the flexibility of your application's data modeling.
Happy coding! 🚀