Associate Custom Objects in HubSpot using Laravel
- Published on
- • 8 mins read•––– views
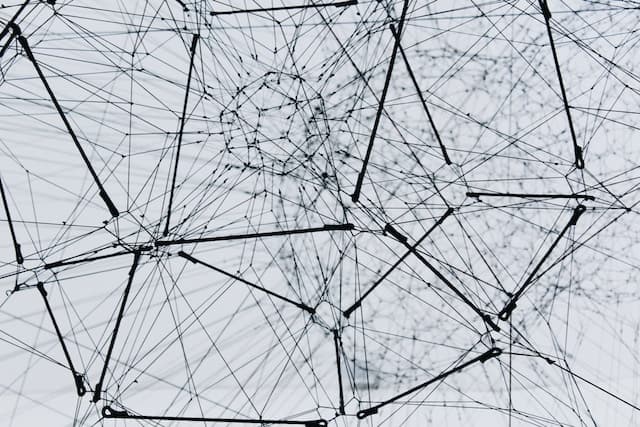
How to Associate Posts with Comments in HubSpot Custom Object using Laravel
In this tutorial, you will learn how to use Laravel to associate posts with comments in HubSpot's custom object using the HubSpot API. We'll create a simple Laravel application that allows you to create posts and associated comments. The post data will be stored in one custom object in HubSpot, while the comment data will be stored in another custom object, and we'll associate the comments with their respective posts.
Introduction
In this tutorial, we will explore how to associate custom objects in HubSpot using Laravel, allowing you to establish relationships between different custom objects in your HubSpot account. We will create a Laravel application that stores and associates comments with posts in HubSpot's custom objects.
Prerequisites
Before starting this tutorial, make sure you have the following:
- A HubSpot developer account.
- Laravel installed on your local machine.
- Basic knowledge of Laravel and PHP.
Step 1: Set Up Your Laravel Project
If you don't have a Laravel project set up yet, follow these steps:
Install Laravel using Composer (if you haven't already):
composer global require laravel/installer
Create a new Laravel project:
laravel new hubspot-associate-posts-comments cd hubspot-associate-posts-comments
Step 2: Install Guzzle HTTP Client
Guzzle is a PHP HTTP client that we'll use to make API requests to HubSpot. Install Guzzle via Composer:
composer require guzzlehttp/guzzle
Step 3: Create the HubspotService Class
Create a new service class HubspotService
that will handle interactions with the HubSpot API to store and associate post and comment data.
Create the HubspotService.php
file in the app/Services
directory and add the following code:
<?php
namespace App\Services;
use GuzzleHttp\Client;
use GuzzleHttp\Exception\GuzzleException;
use Exception;
class HubspotService
{
// Implementation of the storeCustomObject method as shown in the previous code snippet
// ...
}
In this HubspotService
class, we've added a new method associateCommentWithPost
. This method is responsible for associating a comment with a specific post in HubSpot using their custom object IDs. It sends a PATCH request to the HubSpot API to create an association between the post and the comment.
To call the HubspotAssociateObjectEvent
to handle the association between comments and posts, you need to make some changes to the existing code. Let's update the CommentController
to utilize the event for comment-post association:
Step 4: Create the CommentController
Update the CommentController.php
file in the app/Http/Controllers
directory with the following code:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Str;
use App\Http\Requests\CommentRequest;
use App\Events\HubspotCustomObjectEvent;
use App\Events\HubspotAssociateObjectEvent; // Add the HubspotAssociateObjectEvent class here
use App\Services\HubspotService;
class CommentController extends Controller
{
/**
* Handle the incoming request to create a new comment.
*
* @param CommentRequest $request The incoming request containing the comment data.
* @return \Illuminate\Http\JsonResponse
*/
public function store(CommentRequest $request)
{
// Extract the comment data from the incoming request.
$commentData = [
'content' => $request->get('content'),
// Add any other relevant comment properties here...
];
// Create a new HubspotCustomObjectEvent and pass the comment data to be stored in the event payload.
// The 'comments' argument represents the custom object type in HubSpot where the comment data will be stored.
$commentEvent = new HubspotCustomObjectEvent('comments', $commentData);
event($commentEvent);
// Now, associate the comment with the respective post using their custom object IDs.
// For this example, let's assume we have the post ID and comment ID available.
$postId = 'YOUR_POST_ID'; // Replace with the actual post ID
$commentId = 'YOUR_COMMENT_ID'; // Replace with the actual comment ID
// Create a new HubspotAssociateObjectEvent to handle the association between the comment and the post.
// The event payload includes the custom object types and IDs for both comment and post.
$associateEvent = new HubspotAssociateObjectEvent('comments', $commentId, 'posts', $postId);
event($associateEvent);
// Return a response indicating the successful creation of the comment and association.
return response()->json(['message' => 'Comment created and associated with the post successfully!']);
}
}
Step 5: Create the HubspotAssociateObjectEvent
Next, let's create the HubspotAssociateObjectEvent
class that will handle the association between comments and posts in HubSpot.
Create the HubspotAssociateObjectEvent.php
file in the app/Events
directory and add the following code:
<?php
namespace App\Events;
use Illuminate\Foundation\Events\Dispatchable;
use Illuminate\Queue\SerializesModels;
class HubspotAssociateObjectEvent
{
use Dispatchable, SerializesModels;
public $objectType;
public $objectId;
public $associationType;
public $associationId;
/**
* Create a new event instance.
*
* @param string $objectType The custom object type in HubSpot.
* @param string $objectId The ID of the custom object to be associated.
* @param string $associationType The custom object type to associate with.
* @param string $associationId The ID of the custom object to be associated with.
*/
public function __construct(string $objectType, string $objectId, string $associationType, string $associationId)
{
$this->objectType = $objectType;
$this->objectId = $objectId;
$this->associationType = $associationType;
$this->associationId = $associationId;
}
}
Step 6: Update the HubspotService
Now that we have created the HubspotAssociateObjectEvent
, let's update the HubspotService
to handle the comment-post association.
Update the HubspotService.php
file in the app/Services
directory with the following code:
<?php
namespace App\Services;
use GuzzleHttp\Client;
use GuzzleHttp\Exception\GuzzleException;
use Exception;
class HubspotService
{
// ... (Existing code for storeCustomObject)
/**
* Associate a comment with a post in HubSpot's custom objects.
*
* @param string $commentObjectType The custom object type for comments.
* @param string $commentObjectId The ID of the comment custom object.
* @param string $postObjectType The custom object type for posts.
* @param string $postObjectId The ID of the post custom object.
* @throws Exception If any error occurs during the API request or if the response indicates an error.
*/
public function associateCommentWithPost(string $commentObjectType, string $commentObjectId, string $postObjectType, string $postObjectId)
{
$apiUrl = "https://api.hubapi.com/crm/v3/associations/" . $commentObjectType . "/" . $commentObjectId . "/" . $postObjectType . "/" . $postObjectId;
$apiKey = config('services.hubspot.access_token'); // Assuming you have the API key stored in config
$client = new Client();
try {
$response = $client->put($apiUrl, [
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Content-Type' => 'application/json',
'Accept' => 'application/json'
],
]);
$responseBody = $response->getBody()->getContents();
$decodedResponse = json_decode($responseBody);
if (!empty($decodedResponse->status) && $decodedResponse->status == 'error') {
// If the response indicates an error, log the exception.
logException(new Exception($decodedResponse->message ?? ''));
}
} catch (GuzzleException $e) {
// Handle Guzzle related errors and throw a generic Exception with the error message.
throw new Exception("Guzzle Error: " . $e->getMessage());
}
}
}
Step 7: Test the Application
With the changes made to the CommentController
, HubspotAssociateObjectEvent
, and HubspotService
, you can now test the application.
Assuming you have created a post using the /hubspot/post/store
endpoint and obtained the $postId
, you can now create a comment and associate it with the post using the /hubspot/comment/store
endpoint. The event HubspotAssociateObjectEvent
will handle the association between the comment and the post.
Send a POST
request to create a comment and associate it with the post:
POST /hubspot/comment/store
Body: {"content": "This is a comment on the new post!"}
This will create a new comment in HubSpot's custom object representing comments and associate it with the post using the $postId
.
Conclusion
In this tutorial, we learned how to create and associate custom objects in HubSpot using Laravel. We covered the process of creating controllers, requests, events, and services to handle the data flow and association between different custom objects.
By leveraging Laravel's flexibility and the power of the HubSpot API, you can build robust applications that manage and link custom objects efficiently within your HubSpot account. Happy coding!