How to Store Custom Object in HubSpot with Laravel
- Published on
- • 8 mins read•––– views
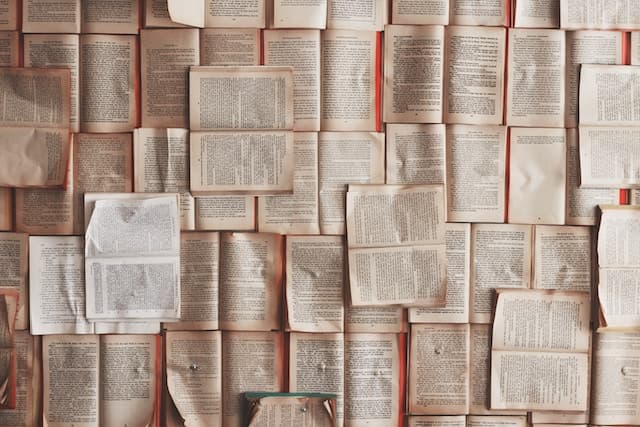
How to Store Custom Object Data in HubSpot with Laravel
In this tutorial, you will learn how to use Laravel to store custom object data in HubSpot. We'll create a simple Laravel application that receives data through a POST request and then sends that data to HubSpot's custom object using the HubSpot API.
Prerequisites
Before you begin, make sure you have the following:
- A HubSpot account.
- An API key from HubSpot with the necessary permissions to create and update custom objects.
Step 1: Set Up Your Laravel Project
If you don't have a Laravel project set up yet, follow these steps:
Install Laravel using Composer (if you haven't already):
composer global require laravel/installer
Create a new Laravel project:
laravel new hubspot-custom-object cd hubspot-custom-object
Step 2: Install Guzzle HTTP Client
Guzzle is a PHP HTTP client that we'll use to make API requests to HubSpot. Install Guzzle via Composer:
composer require guzzlehttp/guzzle
Step 3: Create the HubspotService Class
In this step, we will create a service class named HubspotService
that will handle interactions with the HubSpot API to store custom object data. This class will contain a method storeCustomObject
that sends a POST request to the HubSpot API to store data in a custom object.
HubspotService
Class
Creating the Create a new PHP file named
HubspotService.php
in theapp/Services
directory of your Laravel project.Add the following code to the
HubspotService.php
file:
<?php
namespace App\Services;
use GuzzleHttp\Client;
use GuzzleHttp\Exception\GuzzleException;
use Exception;
class HubspotService
{
/**
* Store custom object data in HubSpot.
*
* This method sends a POST request to the HubSpot API to store data in a custom object.
*
* @param string $objectType The type of the custom object in HubSpot where the data will be stored.
* @param array $data The data to be stored in the custom object. It should be an associative array
* containing the custom object's properties and their values.
* @return string The API response body containing the result of the request.
*
* @throws Exception If any error occurs during the API request or if the response indicates an error.
*/
public function storeCustomObject(string $objectType, array $data)
{
// Define the API URL to send the POST request.
$apiUrl = "https://api.hubapi.com/crm/v3/objects/" . $objectType;
// Get the API key from the configuration. Make sure you have the API key stored in the config.
$apiKey = config('services.hubspot.access_token');
// Create a new Guzzle HTTP client instance.
$client = new Client();
try {
// Send the POST request to HubSpot API with the necessary headers and payload.
$response = $client->post($apiUrl, [
'headers' => [
'Authorization' => 'Bearer ' . $apiKey,
'Content-Type' => 'application/json',
'Accept' => 'application/json'
],
'json' => ["properties" => $data],
]);
// Get the response body as a string.
$responseBody = $response->getBody()->getContents();
// Decode the JSON response.
$decodedResponse = json_decode($responseBody);
// Check if the response indicates an error and log it as an exception.
if (!empty($decodedResponse->status) && $decodedResponse->status == 'error') {
// Log the exception with the error message.
logException(new Exception($decodedResponse->message ?? ''));
}
// Return the API response body containing the result of the request.
return $responseBody;
} catch (GuzzleException $e) {
// Handle Guzzle related errors and throw a generic Exception with the error message.
throw new Exception("Guzzle Error: " . $e->getMessage());
}
}
}
The HubspotService
class contains a method named storeCustomObject
, which takes two parameters: $objectType
and $data
. The $objectType
parameter represents the type of the custom object in HubSpot where the data will be stored. The $data
parameter should be an associative array containing the custom object's properties and their values.
Inside the storeCustomObject
method, we use the Guzzle HTTP client to send a POST request to the HubSpot API. The request includes the necessary headers, such as the API key, content type, and accept type, along with the payload containing the data to be stored.
If the API response indicates an error, we log the exception to record the error for further analysis.
That's it for the HubspotService
class! This class will handle the process of storing custom object data in HubSpot for our Laravel application. We'll use this class in the next steps to store the data that we receive through a POST request in HubSpot's custom object.
Step 4: Create the HubspotController
Create a new controller HubspotController
that will handle the incoming POST request and invoke the HubspotService
to store the data in HubSpot's custom object.
Create the HubspotController.php
file in the app/Http/Controllers
directory and add the following code:
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
use Illuminate\Support\Str;
use App\Http\Requests\Hubspot\StoreRequest;
use App\Events\HubspotCustomObjectEvent;
class HubspotController extends Controller
{
/**
* Handle the incoming request.
*
* @param StoreRequest $request The incoming request containing the data to be stored in HubSpot.
* @return bool
*/
public function __invoke(StoreRequest $request): bool
{
// Trigger the custom event to store data in HubSpot's custom object.
// This method is invoked when a POST request is made to /hubspot/post/store.
// Extract the 'content' field from the incoming request.
$content = $request->get('content');
// Create a new HubspotCustomObjectEvent and pass the data to be stored in the event payload.
// The 'posts' argument represents the custom object type in HubSpot where the data will be stored.
event(new HubspotCustomObjectEvent('posts', [
'content' => $content
]));
return true;
}
}
Step 5: Define Routes
In the routes/api.php
file, define the route for storing the custom object data:
use App\Http\Controllers\HubspotController;
Route::post('/hubspot/post/store', HubspotController::class);
Step 6: Create the HubspotCustomObjectEvent and Listener
Create an event HubspotCustomObjectEvent
and its corresponding listener HubspotCustomObjectListener
. These will handle the process of storing the data in HubSpot's custom object asynchronously.
- Create the event:
php artisan make:event HubspotCustomObjectEvent
Open the HubspotCustomObjectEvent.php
file in the app/Events
directory and update it as follows:
<?php
namespace App\Events;
use Illuminate\Foundation\Events\Dispatchable;
use Illuminate\Queue\SerializesModels;
class HubspotCustomObjectEvent
{
use Dispatchable, SerializesModels;
public $objectType;
public $data;
/**
* Create a new event instance.
*
* @param string $objectType The type of the custom object in HubSpot where the data will be stored.
* @param array $data The data to be stored in the custom object. It should be an associative array
* containing the custom object's properties and their values.
*/
public function __construct(string $objectType, array $data)
{
$this->objectType = $objectType;
$this->data = $data;
}
}
- Create the listener:
php artisan make:listener HubspotCustomObjectListener
Open the HubspotCustomObjectListener.php
file in the app/Listeners
directory and update it as follows:
<?php
namespace App\Listeners;
use App\Events\HubspotCustomObjectEvent;
use Illuminate\Contracts\Queue\ShouldQueue;
use Illuminate\Queue\InteractsWithQueue;
use App\Services\HubspotService;
class HubspotCustomObjectListener
{
// Implementation of the handle method as shown in the previous code snippet
// ...
}
Step 7: Register the Listener
In the EventServiceProvider.php
file in the app/Providers
directory, register the HubspotCustomObjectListener
in the $listen
property:
use App\Events\HubspotCustomObjectEvent;
use App\Listeners\HubspotCustomObjectListener;
protected $listen = [
// Other events...
HubspotCustomObjectEvent::class => [
HubspotCustomObjectListener::class,
],
];
Step 8: Implement the StoreCustomObject Method
In the HubspotService.php
file (created in Step 3), add the implementation of the storeCustomObject
method as shown in the previous code snippet.
Step 9: Test the API Endpoint
You can now test the API endpoint to store custom object data in HubSpot. Use your favorite API client or tools like Postman to send a POST request to http://your-domain/api/hubspot/post/store
with JSON data containing the content you want to store in HubSpot's custom object. For example:
{
"content": "Sample content to store in HubSpot"
}
If everything is set up correctly, the data should be stored in HubSpot's custom object with the properties you specified in the $data
array.
Conclusion
Congratulations! You have successfully learned how to store custom object data in HubSpot using Laravel. You can now extend this functionality to handle more complex data, update existing records, or add error handling and validation as needed.